File Input and Output in C++
Unit 9 File Input and Output in C++
Structure
9.1 Introduction
9.2 Introduction to files and streams in C++
9.3 Character and String input and output to files
9.4 Command Line Arguments and Printer Output
9.1 Introduction
This unit has the following objectives
· To understand how file input output is handled in C++
· To learn how to pass arguments during runtime
· To learn how to handle output to printer
9.2 Introduction to files and streams in C++
File input and output in C++ is handled completely by predefined file and stream related classes. The cin and cout statements which we were using for input from keyboard and output to display screen are also predefined objects of these predefined stream classes.
The header file iostream.h is a header file containing the declarations of these classes which we have been using in all our programs. Similarly other header file fstream.h contains declarations for classes used for disk file I/O.
Let us first understand what is a stream. Streams are name given for flow of data. They are logical abstraction between user and the external device.Each stream is associated and represented through a class in C++. All streams behave in the same way even though they are connected to different devices. This capability allows same functions to be used even though devices are different. The member functions in stream classes contains definitions and procedures for handling a particular kind of data. For example ifstream class is defined for handling input from disk files. So the files in C++ are objects of a particular stream class.
The stream classes are organized in a beautiful hierarchy which is slightly complex. But overview of how they are organized will provide an excellent example of inheritance and help understand files and streams in a better way.
The following figure shows a portion of stream class hierarchy in C++ which we will be using in our programs in this unit
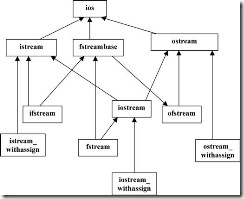
Fig. 9.1: Stream Classes in C++
As shown in the fig. 9.1 all stream classes have been inherited from the base class ios. It contains many constants and member functions common to all kind of input and output. The class istream is derived from ios class which contains all the necessary functions for handling input. Some of the functions such as get(), getline(), read and overloaded extraction (>>) operators are defined in istream class. While ostream class which is also derived from ios class is dedicated for output related functions of all kinds and contains functions such as put() and write and overloaded insertion operator (<<).
The class iostream is inherited from both istream and ostream through multiple inheritance so that other classes can inherit both the functionalities of input and output from it. Three classes istream_withassign, ostream_withassign, iostream_withassign are inherited from istream, ostream, iostream respectively which adds assignment operators to these classes.Cout is an predefined object of the class ostream_withassign class. Cin is an object of the class istream_withassign class.
The class ifstream which is inherited from both istream and fstreambase is used for input files. Similarly, class ofstream which is inherited from both ostream and fstreambase is used for output files. The class fstream inherited from both iostream and fstreambase is used for files that will perform both input and output. All the three classes for file input and output ie fstream, ifstream and ofstream are declared in the header file fstream.h. The file fstream.h also includes iostream.h, so the programs using fstream.h need not explicitly include iostream.h.
Self Assessment questions
1. cin is a predefined object of _____________ class
2. ___________ header file has to be included for file input and output.
3. cout is a predefined object of ____________ class.
9.3 Character and String input and output to files
Let us see some programs that writes and reads characters and strings to text file. To write any data to a file, you should create an object of ofstream class. The function put() is used with the object to write to the file. It however writes one character at a time. The syntax of put() function is :
objectname.put(character variable).
The following program shows the use of put() function:
#include<fstream.h>
# include<string.h>
void main()
{
ofstream outfile(“test.txt”);
char str[ ]=“hello world”;
for (int j=0;j<strlen(str);j++)
outfile.put(str[j]);
}
In the above program we have created an ofstream object named outfile. The constructor of the class is invoked and a text file named test.txt is assigned to the object outfile. A text file is created in the output directory specified in your compiler and if there is already an text file with the same name, then it is overwritten. The contents of the string str is written to the file test.txt. Please note that we have include fstream.h header file.
Since the insertion operator (<<) is overloaded in the class ostream class (ofstream is derived from ostream), it can be used along with the ofstream object to write text directly to the file. The following program shows that
#include<fstream.h>
void main()
{
ofstream outfile(“test.txt”);
outfile<<“This is my first filen”;
outfile<<“Byen”;
}
To read data from any file, you should create an object of ifstream class. The function get() and getline() is used with the object to read the file. The function get() reads one character at a time. The syntax of get() function is :
objectname.get(character variable)
where object is an object of the ifstream class and the character read is stored in the variable.
The following program reads the contents of a text file character by character and prints on the screen.
#include<fstream.h>
#include <conio.h>
void main()
{
ifstream infile("test.txt");
char ch;
clrscr();
while (infile) // infile becomes 0 when eof condition is reached
{infile.get(ch);
cout << ch;}
getch();}
In the above program, we have checked the end of file condition using the objectname itself which becomes zero when end of file is reached. Alternatively, eof() member function is used to check end of file condition. It returns non zero value when end of file is reached and returns zero while it is reading the file.
The getline() function reads line by line as shown in the following program
#include<fstream.h>
#include <conio.h>
void main()
{
ifstream infile("test.txt");
char buffer[80];
clrscr();
while (!infile.eof()) //returns nonzero value when eof condition is reached
{infile.getline(buffer,80);
cout << buffer;}
getch();}
Since the extraction operator (>>) is overloaded in the class istream class (ifstream is derived from istream), it can be used along with the ifstream object to read text. However it reads one word at a time. The following program shows that
#include<fstream.h>
#include <conio.h>
void main()
{
ifstream infile("test.txt");
char buffer[25];
clrscr();
while (infile) //infile becomes 0 when eof condition is reached
{infile>>buffer;
cout << buffer;}
getch();}
While reading files, we have assumed that the files have already been existing on the disk. However it would be wise to include checks that report in case the file you intend to read is not found on the disk. The ios class contains many such status checks. The above program has been rewritten with error check while opening the file.
#include<fstream.h>
#include <conio.h>
void main()
{
ifstream infile("test.txt");
if (!infile) // check for error while opening the file
cout<<”Cannot open file”;
else
{
char buffer[25];
clrscr();
while (infile) //infile becomes 0 when eof condition is reached
{infile>>buffer;
cout << buffer;}
}
getch();}
Self Assessment questions
1. __________ is class object is used to read data from disk files
2. ________ class object is used to write date into disk files
3. __________ functions reads data from files line by line
4. _________ function writes data into files character by character
9.4 Command Line Arguments and Printer Output
Command line arguments are useful to pass arguments to the program while running the program. These arguments can be used within the program and used. Command line arguments are used when invoking the program from DOS. In C++, like C, there are two default variables argc of type int and argv (array of strings) predefined for storing command line arguments which are usually defined as arguments to the main function. The following program shows the implementation of command line arguments and simply displays them.
//comline.cpp
# include <iostream.h>
void main(int argc, char* argv[])
{
cout<<endl<<”argc= ”<<argc;
for (int j=0;j<argc;j++)
cout<<endl<<”Argument ”<<j<<”= ”<<argv[j];
}
If you invoke the above program with the statement
C:/tc> comline sujay pawan vignesh
Then the output will be as follows
argc= 4
Argument0= c:/tc/comline.exe
Argument1= sujay
Argument2= pawan
Argument3= vignesh
As you can see in the output, the argc variable contains the argument count or number of arguments including the filename. Argv contains along with the path of the executable program, the strings passed as arguments. They can be referred by their index number.
Let us see how we can send data to printer. It is similar to sending data to a disk file seen in the last section. The program uses filenames predefined in DOS for various hardware devices. PRN or LPT1 is the filename for the printer which is connected to the first parallel port. LPT2, LPT3 so on can be used if the printer is connected to second, third parallel port respectively.
The following program prints a test message to the printer
//printtest.cpp
# include<fstream.h>
void main()
{ ofstream prnfile(“PRN”);
prnfile<<”This is a test print page”;
}
In the above program we have created a ofstream object and associated it with the printer file name PRN and then flushed the contents to the object. As you can see the code and the way we direct the contents to a stream does not differ. Whether it is sending contents to display screen or printer or disk file, the syntax remains same. That is the beauty of stream class organization hierarchy.
Self Assessment questions
1. __________ is the predefined filename assigned to printer by DOS
2. ________ variable holds the count of command line arguments.
3. ___________ object is created to send any content to printer.
Summary
Disk Input and output is handled is C++ using an hierarchy of stream classes. Files in C++ are objects of classes ifstream for input, ofstream for output and fstream for input as well as output. The class organization and inheritance enables the same syntax and methods to be used irrespective of the hardware device involved in input or output. Cin and cout are predefined objects of stream classes. These classes have several status checks for checking end of file, error conditions etc.
Terminal Questions
1. Write a program that opens a text file named sample.txt and then prints the contents to the printer.
2. Write a program that will be called as mtype.cpp which imitates the type command of the dos. The user should be able to specify the name of the text file along with mtype which is read and the contents displayed on the screen.
3. we can output text to an object of class ofstream using insertion operator << because
a. the ofstream class is a stream
b. the insertion operator works with all classes
c. we are actually outputting to cout
d. the insertion operator is overloaded in ofstream
Answers to SAQs in 9.2
1. istream_withassign
2. fstream.h
3. ostream_withassign
Answers to SAQs in 9.3
1. ifstream
2. ofstream
3. getline()
4. put()
Answers to SAQs in 9.4
1. PRN
2. argc
3. ofstream
Answers to TQs
1. //file2prn.cpp
# include<fstream.h>
void main()
{
char ch;
ifstream infile;
infile.open("sample.txt");
ofstream outfile;
outfile.open("PRN");
while(infile.get(ch))
{ outfile.put(ch);
}
}
2. //mytype.cpp
# include<fstream.h>
# include<process.h>
void main(int argc, char* argv[])
{ if (argc!=2)
{ cerr<<“nFormat: mytype filename”;
exit(-1);
}
char ch;
ifstream infile;
infile.open(arg[1]);
if (!infile)
{ cerr<<“cannot open”<<argv[1];
exit(-1);
}
while(infile)
{infile.get(ch);
cout<<ch;}
}
3. the insertion operator is overloaded in ofstream
Reference Books :
1. E. Balagurusamy, “ Object Oriented Programming with C++”, Tata McGraw-Hill, 2001
2. Robert Lafore, “Object Oriented Programming in C++”, Galgotia Publications, 1994.
3. Herbert Schildt, “The Complete Reference C++”, Tata McGraw-Hill, 2001.
4. Deitel and Deitel, “C++ How to Program”, Pearson Education, 2001
5. Let Us C++, Yashawant Kanitkar, BPB Publications
6. Practical C++ Programming , O’Reilly
7. Beginning C++, Ivor Horton
8. A First book of C++, Gary Bronson
Structure
9.1 Introduction
9.2 Introduction to files and streams in C++
9.3 Character and String input and output to files
9.4 Command Line Arguments and Printer Output
9.1 Introduction
This unit has the following objectives
· To understand how file input output is handled in C++
· To learn how to pass arguments during runtime
· To learn how to handle output to printer
9.2 Introduction to files and streams in C++
File input and output in C++ is handled completely by predefined file and stream related classes. The cin and cout statements which we were using for input from keyboard and output to display screen are also predefined objects of these predefined stream classes.
The header file iostream.h is a header file containing the declarations of these classes which we have been using in all our programs. Similarly other header file fstream.h contains declarations for classes used for disk file I/O.
Let us first understand what is a stream. Streams are name given for flow of data. They are logical abstraction between user and the external device.Each stream is associated and represented through a class in C++. All streams behave in the same way even though they are connected to different devices. This capability allows same functions to be used even though devices are different. The member functions in stream classes contains definitions and procedures for handling a particular kind of data. For example ifstream class is defined for handling input from disk files. So the files in C++ are objects of a particular stream class.
The stream classes are organized in a beautiful hierarchy which is slightly complex. But overview of how they are organized will provide an excellent example of inheritance and help understand files and streams in a better way.
The following figure shows a portion of stream class hierarchy in C++ which we will be using in our programs in this unit
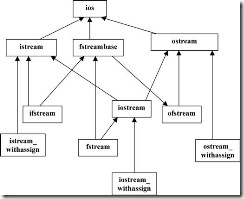
Fig. 9.1: Stream Classes in C++
As shown in the fig. 9.1 all stream classes have been inherited from the base class ios. It contains many constants and member functions common to all kind of input and output. The class istream is derived from ios class which contains all the necessary functions for handling input. Some of the functions such as get(), getline(), read and overloaded extraction (>>) operators are defined in istream class. While ostream class which is also derived from ios class is dedicated for output related functions of all kinds and contains functions such as put() and write and overloaded insertion operator (<<).
The class iostream is inherited from both istream and ostream through multiple inheritance so that other classes can inherit both the functionalities of input and output from it. Three classes istream_withassign, ostream_withassign, iostream_withassign are inherited from istream, ostream, iostream respectively which adds assignment operators to these classes.Cout is an predefined object of the class ostream_withassign class. Cin is an object of the class istream_withassign class.
The class ifstream which is inherited from both istream and fstreambase is used for input files. Similarly, class ofstream which is inherited from both ostream and fstreambase is used for output files. The class fstream inherited from both iostream and fstreambase is used for files that will perform both input and output. All the three classes for file input and output ie fstream, ifstream and ofstream are declared in the header file fstream.h. The file fstream.h also includes iostream.h, so the programs using fstream.h need not explicitly include iostream.h.
Self Assessment questions
1. cin is a predefined object of _____________ class
2. ___________ header file has to be included for file input and output.
3. cout is a predefined object of ____________ class.
9.3 Character and String input and output to files
Let us see some programs that writes and reads characters and strings to text file. To write any data to a file, you should create an object of ofstream class. The function put() is used with the object to write to the file. It however writes one character at a time. The syntax of put() function is :
objectname.put(character variable).
The following program shows the use of put() function:
#include<fstream.h>
# include<string.h>
void main()
{
ofstream outfile(“test.txt”);
char str[ ]=“hello world”;
for (int j=0;j<strlen(str);j++)
outfile.put(str[j]);
}
In the above program we have created an ofstream object named outfile. The constructor of the class is invoked and a text file named test.txt is assigned to the object outfile. A text file is created in the output directory specified in your compiler and if there is already an text file with the same name, then it is overwritten. The contents of the string str is written to the file test.txt. Please note that we have include fstream.h header file.
Since the insertion operator (<<) is overloaded in the class ostream class (ofstream is derived from ostream), it can be used along with the ofstream object to write text directly to the file. The following program shows that
#include<fstream.h>
void main()
{
ofstream outfile(“test.txt”);
outfile<<“This is my first filen”;
outfile<<“Byen”;
}
To read data from any file, you should create an object of ifstream class. The function get() and getline() is used with the object to read the file. The function get() reads one character at a time. The syntax of get() function is :
objectname.get(character variable)
where object is an object of the ifstream class and the character read is stored in the variable.
The following program reads the contents of a text file character by character and prints on the screen.
#include<fstream.h>
#include <conio.h>
void main()
{
ifstream infile("test.txt");
char ch;
clrscr();
while (infile) // infile becomes 0 when eof condition is reached
{infile.get(ch);
cout << ch;}
getch();}
In the above program, we have checked the end of file condition using the objectname itself which becomes zero when end of file is reached. Alternatively, eof() member function is used to check end of file condition. It returns non zero value when end of file is reached and returns zero while it is reading the file.
The getline() function reads line by line as shown in the following program
#include<fstream.h>
#include <conio.h>
void main()
{
ifstream infile("test.txt");
char buffer[80];
clrscr();
while (!infile.eof()) //returns nonzero value when eof condition is reached
{infile.getline(buffer,80);
cout << buffer;}
getch();}
Since the extraction operator (>>) is overloaded in the class istream class (ifstream is derived from istream), it can be used along with the ifstream object to read text. However it reads one word at a time. The following program shows that
#include<fstream.h>
#include <conio.h>
void main()
{
ifstream infile("test.txt");
char buffer[25];
clrscr();
while (infile) //infile becomes 0 when eof condition is reached
{infile>>buffer;
cout << buffer;}
getch();}
While reading files, we have assumed that the files have already been existing on the disk. However it would be wise to include checks that report in case the file you intend to read is not found on the disk. The ios class contains many such status checks. The above program has been rewritten with error check while opening the file.
#include<fstream.h>
#include <conio.h>
void main()
{
ifstream infile("test.txt");
if (!infile) // check for error while opening the file
cout<<”Cannot open file”;
else
{
char buffer[25];
clrscr();
while (infile) //infile becomes 0 when eof condition is reached
{infile>>buffer;
cout << buffer;}
}
getch();}
Self Assessment questions
1. __________ is class object is used to read data from disk files
2. ________ class object is used to write date into disk files
3. __________ functions reads data from files line by line
4. _________ function writes data into files character by character
9.4 Command Line Arguments and Printer Output
Command line arguments are useful to pass arguments to the program while running the program. These arguments can be used within the program and used. Command line arguments are used when invoking the program from DOS. In C++, like C, there are two default variables argc of type int and argv (array of strings) predefined for storing command line arguments which are usually defined as arguments to the main function. The following program shows the implementation of command line arguments and simply displays them.
//comline.cpp
# include <iostream.h>
void main(int argc, char* argv[])
{
cout<<endl<<”argc= ”<<argc;
for (int j=0;j<argc;j++)
cout<<endl<<”Argument ”<<j<<”= ”<<argv[j];
}
If you invoke the above program with the statement
C:/tc> comline sujay pawan vignesh
Then the output will be as follows
argc= 4
Argument0= c:/tc/comline.exe
Argument1= sujay
Argument2= pawan
Argument3= vignesh
As you can see in the output, the argc variable contains the argument count or number of arguments including the filename. Argv contains along with the path of the executable program, the strings passed as arguments. They can be referred by their index number.
Let us see how we can send data to printer. It is similar to sending data to a disk file seen in the last section. The program uses filenames predefined in DOS for various hardware devices. PRN or LPT1 is the filename for the printer which is connected to the first parallel port. LPT2, LPT3 so on can be used if the printer is connected to second, third parallel port respectively.
The following program prints a test message to the printer
//printtest.cpp
# include<fstream.h>
void main()
{ ofstream prnfile(“PRN”);
prnfile<<”This is a test print page”;
}
In the above program we have created a ofstream object and associated it with the printer file name PRN and then flushed the contents to the object. As you can see the code and the way we direct the contents to a stream does not differ. Whether it is sending contents to display screen or printer or disk file, the syntax remains same. That is the beauty of stream class organization hierarchy.
Self Assessment questions
1. __________ is the predefined filename assigned to printer by DOS
2. ________ variable holds the count of command line arguments.
3. ___________ object is created to send any content to printer.
Summary
Disk Input and output is handled is C++ using an hierarchy of stream classes. Files in C++ are objects of classes ifstream for input, ofstream for output and fstream for input as well as output. The class organization and inheritance enables the same syntax and methods to be used irrespective of the hardware device involved in input or output. Cin and cout are predefined objects of stream classes. These classes have several status checks for checking end of file, error conditions etc.
Terminal Questions
1. Write a program that opens a text file named sample.txt and then prints the contents to the printer.
2. Write a program that will be called as mtype.cpp which imitates the type command of the dos. The user should be able to specify the name of the text file along with mtype which is read and the contents displayed on the screen.
3. we can output text to an object of class ofstream using insertion operator << because
a. the ofstream class is a stream
b. the insertion operator works with all classes
c. we are actually outputting to cout
d. the insertion operator is overloaded in ofstream
Answers to SAQs in 9.2
1. istream_withassign
2. fstream.h
3. ostream_withassign
Answers to SAQs in 9.3
1. ifstream
2. ofstream
3. getline()
4. put()
Answers to SAQs in 9.4
1. PRN
2. argc
3. ofstream
Answers to TQs
1. //file2prn.cpp
# include<fstream.h>
void main()
{
char ch;
ifstream infile;
infile.open("sample.txt");
ofstream outfile;
outfile.open("PRN");
while(infile.get(ch))
{ outfile.put(ch);
}
}
2. //mytype.cpp
# include<fstream.h>
# include<process.h>
void main(int argc, char* argv[])
{ if (argc!=2)
{ cerr<<“nFormat: mytype filename”;
exit(-1);
}
char ch;
ifstream infile;
infile.open(arg[1]);
if (!infile)
{ cerr<<“cannot open”<<argv[1];
exit(-1);
}
while(infile)
{infile.get(ch);
cout<<ch;}
}
3. the insertion operator is overloaded in ofstream
Reference Books :
1. E. Balagurusamy, “ Object Oriented Programming with C++”, Tata McGraw-Hill, 2001
2. Robert Lafore, “Object Oriented Programming in C++”, Galgotia Publications, 1994.
3. Herbert Schildt, “The Complete Reference C++”, Tata McGraw-Hill, 2001.
4. Deitel and Deitel, “C++ How to Program”, Pearson Education, 2001
5. Let Us C++, Yashawant Kanitkar, BPB Publications
6. Practical C++ Programming , O’Reilly
7. Beginning C++, Ivor Horton
8. A First book of C++, Gary Bronson
No comments:
Post a Comment