Inheritance and Pointers
Unit 7 Inheritance and Pointers
Structure
7.1 Introduction
7.2 Inheritance in C++
Self Assessment Questions
7.3 Types of Inheritance
Self Assessment Questions
7.4 Introduction to Pointers
Self Assessment Questions
7.5 Objects and Pointers
Self Assessment Questions
Summary
Terminal Questions
7.1 Introduction
This unit has the following objectives
· To understand the concept of inheritance in classes
· To learn different types of interitance
· To learn what are pointers and to use them with default data types as well as classes
·7.2 Inheritance in C++
·Inheritance is a very powerful feature of object oriented programming. It allows reuse of code without modifying the original code. It also allows flexibility to programmer to make modifications to the program without altering the original code which saves debugging and programming time and effort.
Inheritance feature has enabled to distribute class libraries which allows the programmer to use the standard code developed by some another company. Inheritance is the process of creating new classes known as derived classes from the existing or base class. The features of the base class are said to be inherited by the derived class. The child class has all the functionality of the parent class and has additional functionalities of its own.
The data members in a class are usually declared private. But if the class will be inherited by another class, then data members that will be inherited will have to be declared as protected. Protected access specifier allows data members to be accessed by the member functions of inherited class but does not allow to be accessed from external programs. Private members cannot be accessed by the inherited class member functions. The accessibilty of base class members are also dependent on the type of inheritance: private or public. The public inheritance is commonly used which is shown in the program inheritance.cpp discussed next. The types of inheritance are discussed in the next section.
As you can see in the the following diagram, the objects of the derived class can access public members of the base class in addition to the public members of the derived class. The functions of the derived class can access public and protected members of the base class but not the private members of the base class.
Fig. 7.1: Access specifiers in Inheritance (Public)
The following program implements the inheritance. The class manager is inherited from the class employee.
//inheritance.cpp
# include<iostream.h>
# include<string.h>
# include<conio.h>
class employee {
protected:
int empno;
char ename[25];
public:
employee()
{ empno=0;
strcpy(ename,"");
}
employee(int n, char ch[25])
{ empno=n;
strcpy(ename,ch);
}
void display()
{cout<<"Emp Code:"<<empno;
cout<<"Name:"<<ename;
}
};
class manager: public employee {
protected:
float basic;
float hra;
public:
manager():employee()
{ basic=0.0; hra=0.0;}
manager(int n,char ch[25],float i, float j): employee(n,ch)
{ basic=i; hra=j;}
void display()
{ employee::display();
cout<<"Basic"<<basic<<endl;
cout<<"HRA"<<hra<<endl;
}
};
void main()
{
clrscr();
employee e1(106,"amit");
manager m1(205,"pawan",40000.00,5000.00);
e1.display();
m1.display();
getch();
}
In the above program, we have defined a class employee with data members empcode and ename. These have been declared as protected to enable them to be inherited by the derived classes. The employee class also has constructors and a member function display.
The class manager has been derived from the class employee through the statement
class manager: public employee
Here public is a keyword which specifies that the class is derived publicly from class employee. We will see other types of inheritance in the next section. The class manager has basic and hra as data members in addition to ename and empcode
In the class manager also, we have defined constructors and a member function display. While initializing the data members of the manager class, the respective members of the parent class have to be also initialized. We have invoked the constructors of the parent class in the following statements
manager(): employee()
manager(int n,char ch[25],float i, float j): employee(n,ch)
You can also assign default values for the members.
While displaying the contents of the manager class, respective members of the parent class are also displayed. Therefore in the display function of the derived class, we are invoking the display function of the base class also through the statement
employee::display()
Please note here that we are using the class name followed by scope resolution operator and function name to refer to the function. When both the base class and the derived class has a function with same name, the object.functionname statement with the base class object will always access function defined in the base class as the base class does not know anything about the derived classes. If the object is the derived class object, then the the function defined in the derived class will be invoked. This feature is known as function overriding. If the function is not defined in the derived class, then the object will access the function in the parent class.
Self Assessment questions
1. The derived class object can access ______ members of the parent class.
2. The derived class member functions can access _____________ members of the parent class
3. Inheritance allows ___________ of the program code.
7.3 Types of Inheritance
In the previous section, we discussed single inheritance with one base and derived class. We can have several classes derived from a single base class like shown below.
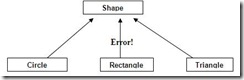
Fig. 7.2: Hierarchial Inheritance example
Inheritance can also be multilevel inheritance where another class is derived from the derived class. In such case the grand child class inherits all the properties of child and parent classes.
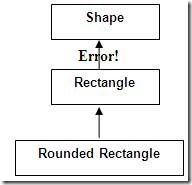
Fig. 7.3: Multilevel Inheritance
The derived class can also have multiple parents which is known as multiple inheritance. Here the child or derived class has two or more parent classes. The child class inherits all the properties of all its parents. Multiple inheritance is implemented same as single inheritance except that both the parent names have to be specified while defining the class. We will discuss multiple inheritance in detail in the next unit.
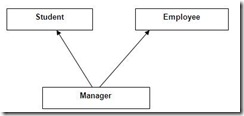
Fig. 7.4: Multiple Inheritance
In the above example we have created a class employee and a student. Manager class is defined from both of these classes. This is useful in instances when you want to create an employee whose educational qualifications need not be stored such as a worker.
We had seen in the inheritance.cpp program, public inheritance. There can also be private inheritance. In the public inheritance, keyword public specifies that objects of derived class can access public member functions of the base class. If the derived class is derived privately then objects of derived class cannot access the public member functions of the base class. Since objects cannot access private or protected members of a class, no members of the base class will be accessible by the objects of the derived class.
The following figure shows accessibility in private inheritance
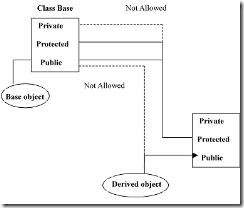
Fig. 7.5: Access Specifiers in Private Inheritance
Self Assessment questions 1. When the derived class has more than one parent it is known as ______
2. In __________ inheritance, objects of derived class cannot access public member functions of the base class
3. A class can be derived further from the derived class. True/false
7.4 Introduction to Pointers
Pointers are sometimes the most confusing concept in C and C++. We will try to demystify the concept here and learn some of the important uses of pointers such as obtaining memory at runtime from the system and creating datastructures such as linked list.
Every variable created in a program has an address which specifies its location in the system’s memory. These addresses can be figured out using & operator or address of operator prefixing the variablename.
Suppose there is an integer variable named num in the program and if you have statement cout<<# , the program will display address of the variable num which will be a hexadecimal number.
Pointers are variables that store address values of other variables. They are defined by prefixing a * to the variable name. The datatype of the pointer variable can be any of the basic or userdefined datatype or even void. A pointer if defined as of a particular datatype, then it can store addresses of variables of that datatype only. However, if the pointer is defined as void then it can store addresses of variables of any datatype. The following statements create a pointer ptr and assigns the address of the integer variable num.
int num=5;
int *ptr;
ptr=#
The variable num can be accessed by two ways now. One through the name num itself and the other through the pointer. The following statement displays the contents of num through the pointer ptr.
cout<<*ptr;
Here * is known as the indirection operator or value of the variable pointed to by the pointer.
If you use cout<<ptr; statement in the program, it will simply display the address of the num or the contents stored in the ptr variable.
One of the important uses of pointers is in getting memory during run time to avoid memory wastage. Let us suppose you want to write a program which accepts a set of numbers from the user and finds the average of the numbers. To store the numbers let us say you would create an array of size 50. The limitation of the program will be that it can work only for maximum of 50 numbers. Also if the user enters numbers less than 50, then the memory is wasted. This usually happens when we do not know the size of the array in advance. The solution to avoid this problem is allocating memory dynamically to the array. New operator in C++ allows this. It is similar to malloc in C. It requests the memory of specific size from the system during runtime and returns the address of the memory location of the first element in the array. To return the memory back to the system, delete operator is used.
The syntax of the new operator is
pointervariable = new datatype[size];
where datatype can be any basic or userdefined
and size is any integer variable.
The syntax of delete operator is
delete pointervariable;
where pointervariable is the pointer containing address returned by the system.
The following program creates a dynamic array and finds the average of the numbers entered by the user.
//dynarray.cpp
#include<iostream.h>
#include<conio.h>
void main()
{
int n,sum=0;
float avg;
int* ptr;
cout<<"enter the size of the array"<<endl;
cin>>n;
ptr=new int[n];
cout<<"enter"<<n<<"numbers";
for(int i=0;i<n;i++)
{cin>>ptr[i];
sum=sum+ ptr[i];}
avg=sum/n;
cout<<"average="<<avg;
delete ptr;
getch();
}
In the above program the statement ptr=new int[n]; asks the system to allocate memory of size n to store integer values and stores the address of the memory allotted in the pointer variable ptr.
Self Assessment questions
1. Pointers are variables that store ____________.
2. ____________ operator can be used to request memory from the system during runtime.
3. The memory allotted dynamically should be returned to the system using ________ operator.
7.5 Objects and Pointers
Pointers can point to userdefined objects as well. If we would like to define a pointer to an object of class employee, we would use the following statement:
employee *ptr;
where ptr is a pointer pointing to object of employee class.
To access the member functions through the pointer there is simple operator -> which is known as membership access operator. This can be used instead of dot operator when using pointers to access the member data or functions.
The following statement accesses member function display of the employee class through the pointer ptr:
ptr->display();
Another important use of pointers is creating datastructures like linked list. Linked list is a dynamic data structure which can be imagined as set of nodes. Every node stores data and link to the next node in the list. The following example implements the node using a structure and linklist using class that stores pointer to the first node in the list.
//linklist.cpp
# include<iostream.h>
# include<conio.h>
struct node
{
int data;
node* next; // pointer to next node
};
class list
{ private:
node *first; // pointer to the first node in the list
public:
list() // no-argument default constructor
{ first=NULL;} // empty list : no first node
void additem(int d) // adds new node to the beginning of the list
{
node* newnode; // node pointer
newnode = new node; // create a new node
newnode->data=d; //assign value
newnode->next=first; // assign pointer to the first
first=newnode; // first now points to new node
}
void display() // displays all nodes
{ node* temp; // pointer to node
temp=first;
while (temp!=NULL)
{ cout<< endl<<temp->data; // print data
temp=temp->next; // points to next node
}
}
};
void main()
{ clrscr();
list list1;
list1.additem(25);
list1.additem(50);
list1.additem(52);
list1.display();
getch();
}
Every object created has a unique pointer created by default known as this pointer.This pointer is a default pointer created for every object that points to the object itself. All the member functions have access to “this pointer”. It is useful to return the object itself after modification. The following program shows the use of this pointer to overload assignment operator =:
# include<iostream.h>
class alpha
{ private:
int data;
public:
alpha() {data=0;}
alpha (int d) {data=d;}
alpha& operator = (alpha& a)
{ data= a.data;
return *this;}
};
void main()
{alpha a1(37), a2;
a2=a1;
a2.display();
}
Self Assessment questions
1. The default pointer that points to the object is known as ____ pointer.
2. The member functions of a class can be accessed through the pointers using ________ operator.
3. The pointer variables can be members of a class. True/False.
Summary
Inheritance allows to create a class known as derived class from a class known as base class and inherit all the properties of the parent class which allows programs to be reused without rewriting entire code. The members that can be inherited have to be declared using protected access specifier. There can be several levels of inheritance and the derived class can be inherited from multiple parents as well.
Pointers are variables that store addresses of other variables. They are helpful mainly is obtaining memory from the system at run time and creating datastructures such as linked lists. Every object has a default pointer known as this pointer.
Terminal Questions
1. Write the output of the following program
# include <iostream.h>
# include<conio.h>
class A {
public:
A() {cout<<"Null Constructor for A" <<endl;}
};
class B: public A {
public:
B() {cout<<"Null Constructor for B" <<endl;}
B(int x) {cout<< "Int constructor for B"<<endl;}
};
class C: public B {
public:
C() {cout<<"Null Constructor for C" <<endl;}
C(int x) : B(x){cout<< "Int constructor for C"<<endl;}
};
void main()
{
clrscr();
A a;
B b;
B bobj(5);
C c;
C cobj(1);
getch();
}
2. Write the output of the following program
# include <iostream.h>
# include<conio.h>
class A {
public:
A() {cout<<"Null Constructor for A" <<endl;}
~A() {cout<< "Destructor for A"<<endl;}
};
class B: public A {
public:
B() {cout<<"Null Constructor for B" <<endl;}
~B() {cout<< "Destructor for B"<<endl;}
};
class C: public B {
public:
C() {cout<<"Null Constructor for C" <<endl;}
~C() {cout<< "Destructor for C"<<endl;}
};
void main()
{
C c;
getch();
}
3. Write another member function deleteitem for the class list to delete the items from the beginning of the list
Answers to SAQs in 7.2
1. public
2. public and protected
3. reusability
Answers to SAQs in 7.3
1. Multiple Inheritance
2. Private Inheritance
3. True
Answers to SAQs in 7.4
1. addresses
2. new
3. delete
Answers to SAQs in 7.5
1. this
2. -> operator
3. True
Answers to TQs
1. Output is:
Null Constructor for A
Null Constructor for A
Null Constructor for B
Null Constructor for A
Int Constructor for B
Null Constructor for A
Null Constructor for B
Null Constructor for C
Null Constructor for A
Int Constructor for B
Int Constructor for C
2. Output is
Null Constructor for A
Null Constructor for B
Null Constructor for C
Destructor for C
Destructor for B
Destructor for A
3. void deleteitem()
{ node* temp;
temp=first;
first=first->next;
delete temp;
}
Structure
7.1 Introduction
7.2 Inheritance in C++
Self Assessment Questions
7.3 Types of Inheritance
Self Assessment Questions
7.4 Introduction to Pointers
Self Assessment Questions
7.5 Objects and Pointers
Self Assessment Questions
Summary
Terminal Questions
7.1 Introduction
This unit has the following objectives
· To understand the concept of inheritance in classes
· To learn different types of interitance
· To learn what are pointers and to use them with default data types as well as classes
·7.2 Inheritance in C++
·Inheritance is a very powerful feature of object oriented programming. It allows reuse of code without modifying the original code. It also allows flexibility to programmer to make modifications to the program without altering the original code which saves debugging and programming time and effort.
Inheritance feature has enabled to distribute class libraries which allows the programmer to use the standard code developed by some another company. Inheritance is the process of creating new classes known as derived classes from the existing or base class. The features of the base class are said to be inherited by the derived class. The child class has all the functionality of the parent class and has additional functionalities of its own.
The data members in a class are usually declared private. But if the class will be inherited by another class, then data members that will be inherited will have to be declared as protected. Protected access specifier allows data members to be accessed by the member functions of inherited class but does not allow to be accessed from external programs. Private members cannot be accessed by the inherited class member functions. The accessibilty of base class members are also dependent on the type of inheritance: private or public. The public inheritance is commonly used which is shown in the program inheritance.cpp discussed next. The types of inheritance are discussed in the next section.
As you can see in the the following diagram, the objects of the derived class can access public members of the base class in addition to the public members of the derived class. The functions of the derived class can access public and protected members of the base class but not the private members of the base class.
The following program implements the inheritance. The class manager is inherited from the class employee.
//inheritance.cpp
# include<iostream.h>
# include<string.h>
# include<conio.h>
class employee {
protected:
int empno;
char ename[25];
public:
employee()
{ empno=0;
strcpy(ename,"");
}
employee(int n, char ch[25])
{ empno=n;
strcpy(ename,ch);
}
void display()
{cout<<"Emp Code:"<<empno;
cout<<"Name:"<<ename;
}
};
class manager: public employee {
protected:
float basic;
float hra;
public:
manager():employee()
{ basic=0.0; hra=0.0;}
manager(int n,char ch[25],float i, float j): employee(n,ch)
{ basic=i; hra=j;}
void display()
{ employee::display();
cout<<"Basic"<<basic<<endl;
cout<<"HRA"<<hra<<endl;
}
};
void main()
{
clrscr();
employee e1(106,"amit");
manager m1(205,"pawan",40000.00,5000.00);
e1.display();
m1.display();
getch();
}
In the above program, we have defined a class employee with data members empcode and ename. These have been declared as protected to enable them to be inherited by the derived classes. The employee class also has constructors and a member function display.
The class manager has been derived from the class employee through the statement
class manager: public employee
Here public is a keyword which specifies that the class is derived publicly from class employee. We will see other types of inheritance in the next section. The class manager has basic and hra as data members in addition to ename and empcode
In the class manager also, we have defined constructors and a member function display. While initializing the data members of the manager class, the respective members of the parent class have to be also initialized. We have invoked the constructors of the parent class in the following statements
manager(): employee()
manager(int n,char ch[25],float i, float j): employee(n,ch)
You can also assign default values for the members.
While displaying the contents of the manager class, respective members of the parent class are also displayed. Therefore in the display function of the derived class, we are invoking the display function of the base class also through the statement
employee::display()
Please note here that we are using the class name followed by scope resolution operator and function name to refer to the function. When both the base class and the derived class has a function with same name, the object.functionname statement with the base class object will always access function defined in the base class as the base class does not know anything about the derived classes. If the object is the derived class object, then the the function defined in the derived class will be invoked. This feature is known as function overriding. If the function is not defined in the derived class, then the object will access the function in the parent class.
Self Assessment questions
1. The derived class object can access ______ members of the parent class.
2. The derived class member functions can access _____________ members of the parent class
3. Inheritance allows ___________ of the program code.
7.3 Types of Inheritance
In the previous section, we discussed single inheritance with one base and derived class. We can have several classes derived from a single base class like shown below.
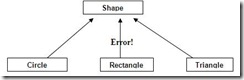
Fig. 7.2: Hierarchial Inheritance example
Inheritance can also be multilevel inheritance where another class is derived from the derived class. In such case the grand child class inherits all the properties of child and parent classes.
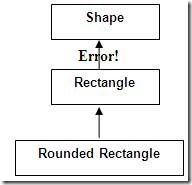
Fig. 7.3: Multilevel Inheritance
The derived class can also have multiple parents which is known as multiple inheritance. Here the child or derived class has two or more parent classes. The child class inherits all the properties of all its parents. Multiple inheritance is implemented same as single inheritance except that both the parent names have to be specified while defining the class. We will discuss multiple inheritance in detail in the next unit.
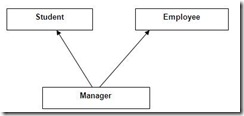
Fig. 7.4: Multiple Inheritance
In the above example we have created a class employee and a student. Manager class is defined from both of these classes. This is useful in instances when you want to create an employee whose educational qualifications need not be stored such as a worker.
We had seen in the inheritance.cpp program, public inheritance. There can also be private inheritance. In the public inheritance, keyword public specifies that objects of derived class can access public member functions of the base class. If the derived class is derived privately then objects of derived class cannot access the public member functions of the base class. Since objects cannot access private or protected members of a class, no members of the base class will be accessible by the objects of the derived class.
The following figure shows accessibility in private inheritance
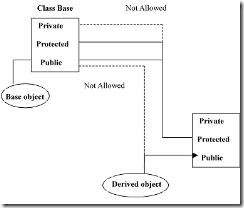
Fig. 7.5: Access Specifiers in Private Inheritance
|
2. In __________ inheritance, objects of derived class cannot access public member functions of the base class
3. A class can be derived further from the derived class. True/false
7.4 Introduction to Pointers
Pointers are sometimes the most confusing concept in C and C++. We will try to demystify the concept here and learn some of the important uses of pointers such as obtaining memory at runtime from the system and creating datastructures such as linked list.
Every variable created in a program has an address which specifies its location in the system’s memory. These addresses can be figured out using & operator or address of operator prefixing the variablename.
Suppose there is an integer variable named num in the program and if you have statement cout<<# , the program will display address of the variable num which will be a hexadecimal number.
Pointers are variables that store address values of other variables. They are defined by prefixing a * to the variable name. The datatype of the pointer variable can be any of the basic or userdefined datatype or even void. A pointer if defined as of a particular datatype, then it can store addresses of variables of that datatype only. However, if the pointer is defined as void then it can store addresses of variables of any datatype. The following statements create a pointer ptr and assigns the address of the integer variable num.
int num=5;
int *ptr;
ptr=#
The variable num can be accessed by two ways now. One through the name num itself and the other through the pointer. The following statement displays the contents of num through the pointer ptr.
cout<<*ptr;
Here * is known as the indirection operator or value of the variable pointed to by the pointer.
If you use cout<<ptr; statement in the program, it will simply display the address of the num or the contents stored in the ptr variable.
One of the important uses of pointers is in getting memory during run time to avoid memory wastage. Let us suppose you want to write a program which accepts a set of numbers from the user and finds the average of the numbers. To store the numbers let us say you would create an array of size 50. The limitation of the program will be that it can work only for maximum of 50 numbers. Also if the user enters numbers less than 50, then the memory is wasted. This usually happens when we do not know the size of the array in advance. The solution to avoid this problem is allocating memory dynamically to the array. New operator in C++ allows this. It is similar to malloc in C. It requests the memory of specific size from the system during runtime and returns the address of the memory location of the first element in the array. To return the memory back to the system, delete operator is used.
The syntax of the new operator is
pointervariable = new datatype[size];
where datatype can be any basic or userdefined
and size is any integer variable.
The syntax of delete operator is
delete pointervariable;
where pointervariable is the pointer containing address returned by the system.
The following program creates a dynamic array and finds the average of the numbers entered by the user.
//dynarray.cpp
#include<iostream.h>
#include<conio.h>
void main()
{
int n,sum=0;
float avg;
int* ptr;
cout<<"enter the size of the array"<<endl;
cin>>n;
ptr=new int[n];
cout<<"enter"<<n<<"numbers";
for(int i=0;i<n;i++)
{cin>>ptr[i];
sum=sum+ ptr[i];}
avg=sum/n;
cout<<"average="<<avg;
delete ptr;
getch();
}
In the above program the statement ptr=new int[n]; asks the system to allocate memory of size n to store integer values and stores the address of the memory allotted in the pointer variable ptr.
Self Assessment questions
1. Pointers are variables that store ____________.
2. ____________ operator can be used to request memory from the system during runtime.
3. The memory allotted dynamically should be returned to the system using ________ operator.
7.5 Objects and Pointers
Pointers can point to userdefined objects as well. If we would like to define a pointer to an object of class employee, we would use the following statement:
employee *ptr;
where ptr is a pointer pointing to object of employee class.
To access the member functions through the pointer there is simple operator -> which is known as membership access operator. This can be used instead of dot operator when using pointers to access the member data or functions.
The following statement accesses member function display of the employee class through the pointer ptr:
ptr->display();
Another important use of pointers is creating datastructures like linked list. Linked list is a dynamic data structure which can be imagined as set of nodes. Every node stores data and link to the next node in the list. The following example implements the node using a structure and linklist using class that stores pointer to the first node in the list.
//linklist.cpp
# include<iostream.h>
# include<conio.h>
struct node
{
int data;
node* next; // pointer to next node
};
class list
{ private:
node *first; // pointer to the first node in the list
public:
list() // no-argument default constructor
{ first=NULL;} // empty list : no first node
void additem(int d) // adds new node to the beginning of the list
{
node* newnode; // node pointer
newnode = new node; // create a new node
newnode->data=d; //assign value
newnode->next=first; // assign pointer to the first
first=newnode; // first now points to new node
}
void display() // displays all nodes
{ node* temp; // pointer to node
temp=first;
while (temp!=NULL)
{ cout<< endl<<temp->data; // print data
temp=temp->next; // points to next node
}
}
};
void main()
{ clrscr();
list list1;
list1.additem(25);
list1.additem(50);
list1.additem(52);
list1.display();
getch();
}
Every object created has a unique pointer created by default known as this pointer.This pointer is a default pointer created for every object that points to the object itself. All the member functions have access to “this pointer”. It is useful to return the object itself after modification. The following program shows the use of this pointer to overload assignment operator =:
# include<iostream.h>
class alpha
{ private:
int data;
public:
alpha() {data=0;}
alpha (int d) {data=d;}
alpha& operator = (alpha& a)
{ data= a.data;
return *this;}
};
void main()
{alpha a1(37), a2;
a2=a1;
a2.display();
}
Self Assessment questions
1. The default pointer that points to the object is known as ____ pointer.
2. The member functions of a class can be accessed through the pointers using ________ operator.
3. The pointer variables can be members of a class. True/False.
Summary
Inheritance allows to create a class known as derived class from a class known as base class and inherit all the properties of the parent class which allows programs to be reused without rewriting entire code. The members that can be inherited have to be declared using protected access specifier. There can be several levels of inheritance and the derived class can be inherited from multiple parents as well.
Pointers are variables that store addresses of other variables. They are helpful mainly is obtaining memory from the system at run time and creating datastructures such as linked lists. Every object has a default pointer known as this pointer.
Terminal Questions
1. Write the output of the following program
# include <iostream.h>
# include<conio.h>
class A {
public:
A() {cout<<"Null Constructor for A" <<endl;}
};
class B: public A {
public:
B() {cout<<"Null Constructor for B" <<endl;}
B(int x) {cout<< "Int constructor for B"<<endl;}
};
class C: public B {
public:
C() {cout<<"Null Constructor for C" <<endl;}
C(int x) : B(x){cout<< "Int constructor for C"<<endl;}
};
void main()
{
clrscr();
A a;
B b;
B bobj(5);
C c;
C cobj(1);
getch();
}
2. Write the output of the following program
# include <iostream.h>
# include<conio.h>
class A {
public:
A() {cout<<"Null Constructor for A" <<endl;}
~A() {cout<< "Destructor for A"<<endl;}
};
class B: public A {
public:
B() {cout<<"Null Constructor for B" <<endl;}
~B() {cout<< "Destructor for B"<<endl;}
};
class C: public B {
public:
C() {cout<<"Null Constructor for C" <<endl;}
~C() {cout<< "Destructor for C"<<endl;}
};
void main()
{
C c;
getch();
}
3. Write another member function deleteitem for the class list to delete the items from the beginning of the list
Answers to SAQs in 7.2
1. public
2. public and protected
3. reusability
Answers to SAQs in 7.3
1. Multiple Inheritance
2. Private Inheritance
3. True
Answers to SAQs in 7.4
1. addresses
2. new
3. delete
Answers to SAQs in 7.5
1. this
2. -> operator
3. True
Answers to TQs
1. Output is:
Null Constructor for A
Null Constructor for A
Null Constructor for B
Null Constructor for A
Int Constructor for B
Null Constructor for A
Null Constructor for B
Null Constructor for C
Null Constructor for A
Int Constructor for B
Int Constructor for C
2. Output is
Null Constructor for A
Null Constructor for B
Null Constructor for C
Destructor for C
Destructor for B
Destructor for A
3. void deleteitem()
{ node* temp;
temp=first;
first=first->next;
delete temp;
}
No comments:
Post a Comment